Our Blogs
Mobile application development is a set of processes and procedures involved in writing application software for small, handheld or wireless computing devices such as smart phones, PDAs, EDAs or tablets.
In Mobile App development process, Mobile User Interface (UI) Design is also an essential part considering constraints & contexts, screen, input and mobility as outlines for design.
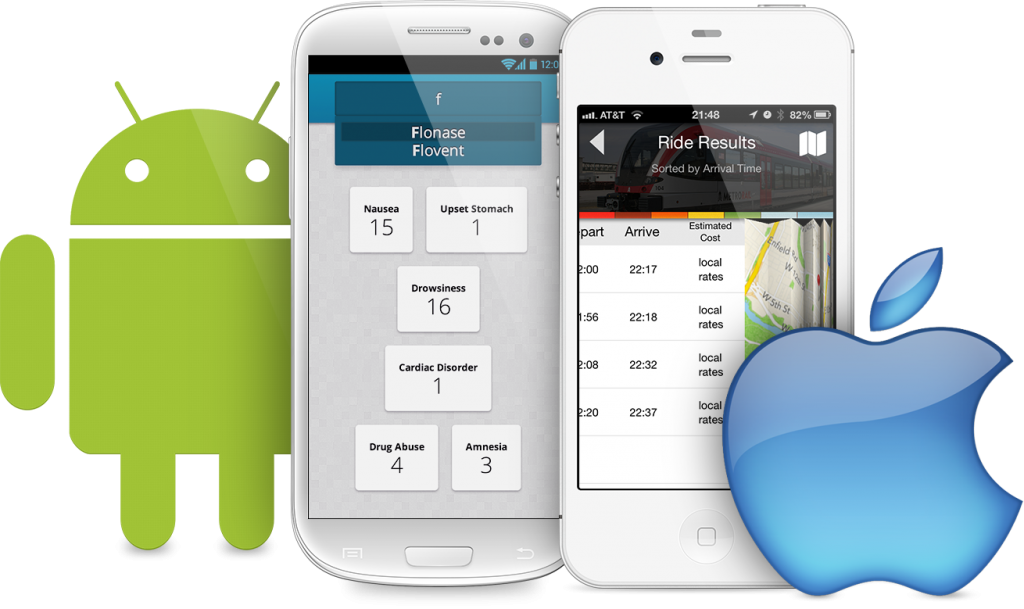
One critical difference between Mobile application development and traditional software development, is that mobile applications (apps) are often written specifically to take advantage of the unique features a particular mobile device offers. So, the development should be centric on optimum performance for a given device, even when application is interacting with external applications.
In such a scenario (interaction with external world) REST API is the best option. REST stands for Representational State Transfer & it is a simple stateless architecture and a communication approach that generally runs over HTTP.
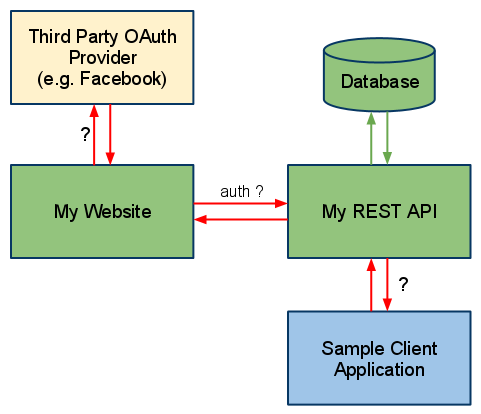
REST is preferred over SOAP (Simple Object Access Protocol) for development of web services as the latter is quite heavyweight and consumes comparatively greater bandwidth. Owing to its decoupled architecture and lighter weight communications, REST usage is very prevalent in mobile applications. It is a better fit for use over the Internet and easily binds with cloud-based APIs like those of Amazon, Microsoft, and Google.
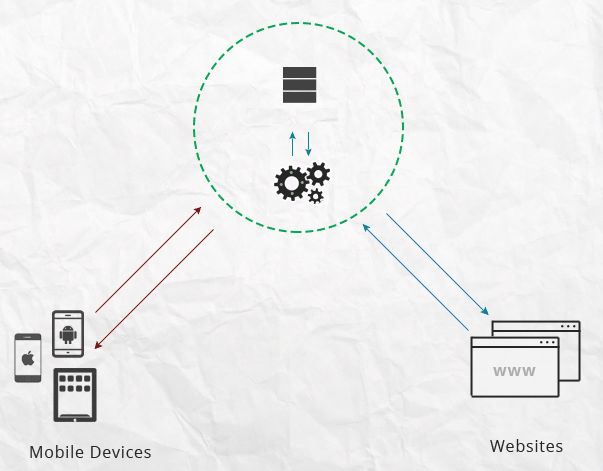
Here is an example to ” Create REST API client Async in android ”
1. This is AsyncTask which will run in background with affecting the UI.
import java.io.BufferedInputStream; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import android.os.AsyncTask; public class CallRestApi extends AsyncTask{ public AsyncResponse delegate=null; public CallRestApi(AsyncResponse asyncResponse) { delegate = asyncResponse;//Assigning call back interfacethrough constructor } @Override protected void onPreExecute() { // if you want, start progress dialog here } @Override protected String doInBackground(String… params) { String urlString = params[0]; String resultToDisplay = “”; InputStream in = null; try { URL url = new URL(urlString); HttpURLConnection urlConnection = (HttpURLConnection) url .openConnection(); in = new BufferedInputStream(urlConnection.getInputStream()); resultToDisplay = convertStreamToString(in); in.close(); } catch (Exception e) { System.out.println(e.getMessage()); return e.getMessage(); } return resultToDisplay; } @Override protected void onPostExecute(String result) { // if you started progress dialog dismiss it here delegate.processFinish(result); } private static String convertStreamToString(InputStream is) { BufferedReader reader = new BufferedReader(new InputStreamReader(is)); StringBuilder sb = new StringBuilder(); String line = null; try { while ((line = reader.readLine()) != null) { sb.append(line + “\n”); } } catch (IOException e) { e.printStackTrace(); } finally { try { is.close(); } catch (IOException e) { e.printStackTrace(); } } return sb.toString(); } }
2. Create Interface for getting result. This is for getting AsyncResponse in the activity class public interface AsyncResponse { void processFinish(Object output); }
3. Call REST API client from activity class
private void functionName(String newUrl) { CallRestApi client = new CallRestApi(new AsyncResponse() { @Override public void processFinish(Object output) { String responseResult = ((String) output); }); } try { client.execute(new String[] { newUrl }); } catch (Exception e) { e.printStackTrace(); } }Enjoy 🙂 Avinash That is all for this article, in case you need Salesforce Implementation Services for any Salesforce related work, then please feel free to reach out to sales@girikon.com